Nov 26, 2023 By Team YoungWonks *
What is a HashMap in Python?
A HashMap in Python is essentially a data structure known as a dictionary. Similar to a HashMap in Java, a Python dictionary stores key-value pairs where each unique key maps to a value. Keys are used to retrieve their corresponding values, facilitating efficient data lookups. These dictionaries are mutable, meaning they can be modified after their creation by adding, updating, or removing key-value pairs.
What is the purpose of a HashMap?
In Python, a HashMap can be compared to a dictionary. It allows us to store key-value pairs where the key is unique. The purpose of HashMap or dictionary in Python is to provide fast access to data based on a unique key. It employs a technique called hashing, where a key's value is converted into a certain index. This calculated index is used for data retrieval, making HashMaps highly efficient for lookups and modifications.
What are the advantages of a HashMap?
HashMap in Python offers several significant advantages.
- HashMaps in Python contribute to cleaner code, increased efficiency, and more straightforward data manipulation.
- HashMaps can store data in a pair form (key-value), making them particularly useful for problems where associations are important.
- It allows for fast data access, as it uses keys for retrieving the values, which often leads to a constant-time complexity for lookup operations.
- It ensures that keys are unique and therefore, eliminates any chances of data redundancy.
What is the difference between a HashMap and a dictionary in Python?
The following are some of the differences between a HashMap and a dictionary:
- A HashMap is a data structure used in Java, not Python. It allows you to store key-value pairs and offers methods to perform operations like add, remove, and retrieve based on the key. On the other hand, a dictionary is a built-in data type in Python that also stores key-value pairs.
- In a HashMap, keys are not ordered, while in a Python dictionary, as of Python 3.7, keys maintain their insertion order.
- HashMap in Java does not allow duplicate keys but allows one null key and multiple null values. Similarly, Python dictionaries do not allow duplicate keys, but they allow both keys and values to be of any data type, including None.
- HashMap relies on the hashCode and equals methods for storing and retrieving data. Python dictionary, on the other hand, uses hash tables which internally use the hash function to calculate the location of data.
How to create a HashMap in Python?
The syntax to create a HashMap in Python, or as it's commonly known, a dictionary, is straightforward and intuitive. You define a dictionary by enclosing a comma-separated list of key-value pairs in curly braces {}. A colon : separates each key from its associated value. Here's a simple example:
Creating a dictionary / hashmap in Python
fruit_dict = {'apple': 1, 'banana': 2, 'orange': 3}
In the above example, 'apple', 'banana', and 'orange' are the keys, and 1, 2, and 3 are their corresponding values. You can use these keys to access their respective values in your dictionary.
How to use a HashMap in Python?
Using a HashMap (or a dictionary) in Python is straightforward. You can access the value of a specific key by referencing it within square brackets []. If the key exists in the dictionary, Python returns the corresponding value. If the key doesn't exist, Python throws a KeyError. To avoid this, you can use the get() method, which returns None if the key doesn't exist. Below is an example:
Python code to access values of a dictionary / hashmap
print(fruit_dict['banana']) # Output: 2
print(fruit_dict['grape']) # This line would throw KeyError
print(fruit_dict.get('grape')) # Output: None
In this example, you can see how we access the value associated with the key 'banana'. Also, we demonstrate how to prevent a KeyError when trying to access a non-existent key by using the get() method.
How to put multiple values for one key in a Python HashMap?
In Python, you can assign multiple values to a single key in a HashMap (also referred to as a dictionary) by storing the values in a list or a tuple. Here's an example:
Put multiple values for one key
my_dict["key"] = ["value1", "value2", "value3"]
In this example, the values "value1", "value2", and "value3" are stored in a list and assigned to the key "key". Now, if you want to add a new value to this key, you will append the value to the list associated with the key:
my_dict["key"].append("value4")
How to make a list into a HashMap in Python?
Here is a brief guide on how to convert a list into a Python hash map (dictionary):
This dictionary allows you to quickly lookup the index of any item in the original list.
Applications of HashMaps in Python
The following are some of the applications of HashMaps in Python:
Tracking Unique Items and Counting Frequencies
Hashmaps, also known as dictionaries in Python, are used to track unique items across a dataset. This is due to its property of maintaining only distinct keys. They are extensively used to count the frequency of elements in a list or any other iterable in Python.
The following is a simple Python program that demonstrates tracking unique items using dictionaries / hashmaps.
In this program, we use the get method of the dictionary. This method returns the value for a key if it exists in the dictionary, else it returns a default value, in this case, 0. This allows us to track unique items and their counts.
Caching using HashMaps
Hashmaps can be used to implement cache to increase the performance of Python programs by storing the results of expensive function calls.
The following Python code demonstrates the concept of caching using dictionaries. This is a simple example of a Fibonacci sequence where previous results are stored in a dictionary to avoid redundant computations:
In this program, cache is a dictionary that maps an input number n to its Fibonacci number. The fibonacci function first checks if the Fibonacci number for n is in the cache. If it is, the function returns it. If it's not, the function calculates it, stores it in the cache, and then returns it.
Data Retrieval using HashMaps
They allow fast data retrieval when the key is known, facilitating quick search operations. Python dictionaries offer fast data retrieval because they utilize a technique known as hashing. In a dictionary, each unique key is associated with a value, forming a key-value pair. When you need to access a value, instead of searching through every key-value pair (which would take linear time), Python uses the key to generate a unique hash. This hash directly maps to a location in memory where the corresponding value is stored. Due to this direct access, dictionaries can retrieve data in constant time, irrespective of their size, thus making them exceptionally efficient for tasks involving frequent data lookups.
Implementing Associative Arrays using HashMaps
In Python, dictionaries are a built-in data type used to create associative arrays. An associative array is a collection of key-value pairs where each key is unique. Here's a simple example of how to implement an associative array using a dictionary in Python:
In this example, the student names are the keys, and their grades are the values. You can use the keys to directly access their corresponding values.
Grouping Data using HashMaps
Hashmaps can be used for grouping data, which can be particularly useful in data analysis tasks.
Python dictionaries are a versatile tool for grouping data. Here's a simple example:
In this example, we've grouped different fruits based on their color using a Python dictionary.
Frequently asked interview questions related to HashMaps in Python
In this section, we are going to discuss some of the interview questions in the field of computer science that directly or indirectly linked to hash maps or dictionaries.
What are the algorithms in which a HashMap can be used as a data structure?
Hashmaps are a crucial data structure used in various algorithms, offering efficient search, insert, and delete operations. Here are a few examples:
- Frequency Counting: Hashmaps are often used to count the frequency of elements in a collection. This is commonly employed in problems where you need to find the most frequent element, or sort elements by frequency.
- Subarray Sum: In problems related to finding subarrays with a given sum, hashmaps can store the cumulative sum at each index. This allows for a quick lookup to check if a sum exists within the array.
- Duplicate Detection: A HashMap can be used to detect duplicates in a collection. Each element is added to the HashMap as a key, and if an element already exists as a key, it is a duplicate.
- Group Anagrams: HashMaps can group anagrams together by storing sorted versions of words as keys and original words as values in the map. The values with the same key are anagrams of each other.
Remember, the key concept behind these algorithms is the ability of a HashMap to perform constant time complexity operations, making it highly efficient for large datasets.
What is the application of HashMap in Data Science and Machine Learning?
In the realm of data science and machine learning, hash maps (also known as hash tables) are integral for managing and processing large-scale data efficiently. These data structures allow for constant-time complexity in accessing, inserting, and deleting data, making them highly efficient. Hashmaps are particularly useful in tasks such as feature extraction, where unique features are mapped to specific numerical indices, saving computational time and resources in the process. Moreover, they play a crucial role in handling categorical data, where unique categories can be mapped to specific numerical values for processing in machine learning algorithms.
How to implement a Linked List in Python using dictionaries?
To implement a linked list in Python using dictionaries, we start by creating a node. Each node is a dictionary with two keys: 'data' and 'next'. 'Data' stores the value of the node and 'next' points to the next node in the list.
First, let's initialize a linked list with one node.
linked_list = {'data': 'Node1', 'next': None}
This creates a linked list with one node ("Node1"). The 'next' field is None, indicating the end of the list.
To add another node to the list, we create a new dictionary and set the 'next' field of the first node to point to the new node.
linked_list['next'] = {'data': 'Node2', 'next': None}
Now, our linked list has two nodes, 'Node1' pointing to 'Node2'.
You can continue this process to add more nodes to the linked list. To traverse the linked list, you can create a loop that follows the 'next' fields from one dictionary to the next until it reaches None.
How to avoid collisions in Python dictionaries?
In Python, dictionary data type is designed to handle data in pairs of keys and values. A key in a dictionary must be unique, therefore, to avoid collisions in Python dictionaries, ensure that keys are not duplicated. If a situation arises where you need to assign a new value to an existing key, Python will overwrite the old value with the new one. Hence, if you want to keep all values, consider using lists or another container type as dictionary values. Example:
my_dict = {}
my_dict['key1'] = ['value1']
my_dict['key1'].append('value2')
print(my_dict) # Output: {'key1': ['value1', 'value2']}
In the example above, key1 has two values, value1 and value2, and there is no collision, as both values are preserved.
What are the methods that can be used on a hash map in Python?
In Python, a dict object (similar to a hashmap in other languages) provides several methods for interacting with the key-value pairs it contains. Here are few important ones:
- keys(): Returns a new view of the dictionary's keys.
- values(): Returns a new view of the dictionary's values.
- items(): Returns a new view of the dictionary's items (key, value pairs).
- get(key, [default]): Returns the value for key if key is in the dictionary, else default.
- setdefault(key, [default]): If key is in the dictionary, return its value. If not, insert key with a value of default and return default.
- update([other]): Update the dictionary with the key/value pairs from other, overwriting existing keys.
- pop(key, [default]): Remove specified key and return the corresponding value.
- clear(): Remove all items from the dictionary.
How to become a Python developer?
To become a Python developer, you should focus on mastering the following concepts and skills:
- Understanding the Python syntax and built-in functions: This includes knowledge of Python's def keyword, which is used to define functions, and how to use str for converting objects into strings.
- Familiarity with Python data types: Grasp the usage and operation of lists, tuples, sets, and dictionaries. This includes understanding dict.items and dict.values for manipulating and accessing dictionary data.
- Working with the init function: Also known as the constructor, this special method is used for initializing newly created objects.
- Mastering Python's lambda functions: These are small anonymous functions used where function objects are required.
- Flask / Django: Learn Flask / Django, the high-level Python web frameworks that enable rapid development and pragmatic design.
- Understanding the concept of hash value: Learn how Python uses hash values for quick lookups and when hashing is useful.
- Grasping the concept of stack: A stack is a collection of items where the addition of new items and the removal of existing items always takes place at the same end.
- Pandas: Get a grasp of Pandas, the powerful python data analysis tool, which will enable you to manipulate and analyze complex datasets.
- Familiarity with Python Libraries: Libraries such as NumPy, Matplotlib, and others are essential tools for Python developers.
- Learning DevOps: Though not directly related to Python, familiarity with DevOps practices will make you a more versatile developer.
- Completing a Python tutorial: This is a step towards practicing and refining your Python skills.
- Understanding the boolean values: Learn the use and importance of the True and False keywords in Python, which represent boolean true and false values.
- Learning how to create and use parameters: Parameters are used in functions to accept inputs and produce outputs.
Remember that becoming a successful Python developer requires constant learning and practice. Keep coding and stay curious.
Coding Classes for Kids
At YoungWonks, we provide an extensive range of Coding Classes for Kids, designed to foster creativity, critical thinking, and problem-solving skills. One of our key offerings is the Python Coding Classes for Kids where we cover important topics such as Python HashMap. In addition to Python, we also offer Full Stack Web Development Classes, equipping young learners with the skills they need to stay competitive in today's rapidly evolving technological landscape.
Check out our YouTube video to revise Python HashMaps.
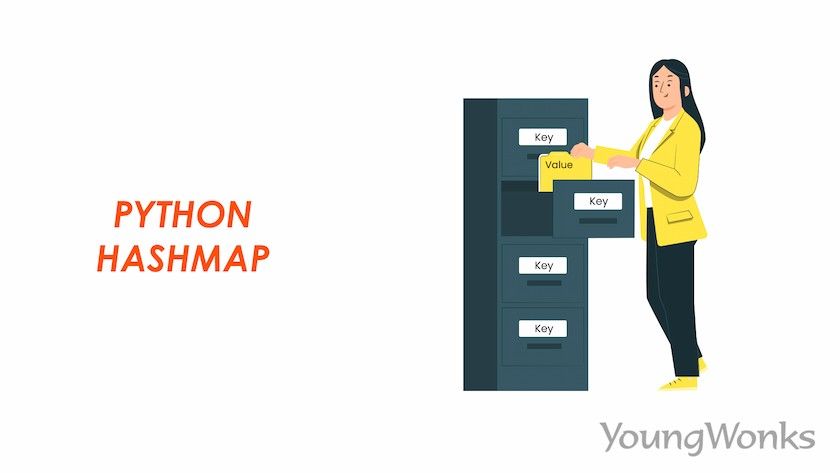
*Contributors: Written by Rohit Budania; Lead image by Shivendra Singh; YouTube video by Kashif Arbaz